Programmatically adding new customers to the Magento
Us developers love adding things programmatically. Even though you can create a new customer through a signup form, or via admin interface, in some cases, that might take too long.
If you need a bunch of customers assigned to different groups, from different countries, you might be better off doing this from the code.
For starters, let’s add a customer with some basic information.
<?php $websiteId = Mage::app()->getWebsite()->getId(); $store = Mage::app()->getStore(); $customer = Mage::getModel("customer/customer"); $customer ->setWebsiteId($websiteId) ->setStore($store) ->setFirstname('Mage') ->setLastname('Spider') ->setEmail('info@magespider.com') ->setPassword('password'); try{ $customer->save(); } catch (Exception $e) { Zend_Debug::dump($e->getMessage()); } ?>As we can see, the code above adds a customer with only first and last names, email and password set. This sometimes might be enough, but we can do more. You could add middle name, assign the customer to a specific customer group, even add prefixes and suffixes to his/hers name.
<?php $customer ->setWebsiteId($websiteId) ->setStore($store) ->setGroupId(2) ->setPrefix('Sir') ->setFirstname('John') ->setMiddleName('2') ->setLastname('Doe') ->setSuffix('II') ->setEmail('info@magespider.com') ->setPassword('somepassword'); ?>Note that the setCountryId needs country code as value (you could find out these values by inspecting ‘Country’ input field on customer creation page in administration). Same goes for setGroupId method above, you need an ID of a customer group, which is easiest to find out by inspecting elements. I hope most of the code is self-explanatory, since we know what fields are required when adding a customer from the administration. The thing you should keep an eye on are required fields. In case you would need multiple customers to be added, you could place the part of the code in a loop, and create them within seconds.
Pritesh
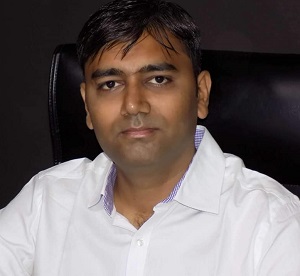
Pritesh Pethani